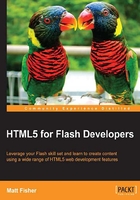
Syntax differences
Now that we have some media to work with and the browser tools at our disposal, let's start some more toying with JavaScript and compare its syntax to what you already know in ActionScript 3.
Variables
Unlike variables declared within ActionScript 3, JavaScript variables are not strictly typed. This takes the familiar ActionScript 3 variable declaration from:
var myVariable:String = 'abc123';
to a simpler syntax within JavaScript which looks as follows:
var myVariable = 'abc123';
This lack of strict typing is referred to as dynamic typing. Variables in JavaScript can be used as any type at any time. Consider this example:
var exampleVar; // A undefined variable exampleVar = "Some example text"; // Variable is now a String exampleVar = 12345; // Variable is now a Number
Dynamic typing allows code to be writing faster by requiring less input from the developer, but this development ease comes at the cost of debugging large applications. ActionScript 3's strict typing allows the compiler to catch issues even before exporting a new version of your application. JavaScript will not do this natively, and it is probably one of the biggest complaints most developers with prior ActionScript 3 experience have when using the language.
Variable type conversion
Although variables in JavaScript are not strictly typed, there are methods to ensure that variable data is in correct form for the desired action. Type conversion can be used on variables to ensure they are formatted properly:
myString = String('12345'); // Convert to a String myBoolean = Boolean('true'); // Convert to Boolean myNumber = Number('12345'); // Convert to Number
Conditions and loops
We will cover these two aspects together as the syntax for conditions and loops in JavaScript are almost the same to what you are used to in ActionScript 3. If
, if... else
, and if... else if
conditions are no different than that in ActionScript:
if(cats > dogs) { // Code for cat people... } else if (cats < dogs) { // Code for dog people... } else { // Code for everyone else... }
Also, the switch
statements can be used and just like if
statements; the syntax is exactly the same as that in ActionScript:
switch(animal) { case 'cat': // Code for cat people... break; case 'dog': // Code for dog people... break; default: // Code for everyone else... }
Loops are no different to their counterparts in ActionScript. Consider these for
and while
loops:
for(var n = 0; n < myArray.length; n++) { // Code within loop... } while(n < 100) { // Code within loop... } do { // Code within loop... } while(n < 100);
Functions
As in ActionScript 3, functions in JavaScript are blocks of code encased within curly braces ({ }
). Every function is associated with a keyword that is used to call the function and run the encased code within it. As usual, functions may return values back to the point where the call was originally made. This is accomplished using the return
statement.
The syntax of JavaScript function is very similar to ActionScript functions but is without the need for strict typing of expected parameters and the function return types. As a Flash developer, your ActionScript 3 functions probably looked something like the follows:
function getCoffee (owner:String, milks:int, sugars:int):void { // Code... }
This syntax can be easily converted to JavaScript just by removing the variable and return type declarations so that the same function in JavaScript can be written as follows:
function getCoffee (owner, milks, sugars) { // Code... }
Objects
Technically, everything declared in JavaScript is an object, however, time will come when you will need to create your own custom objects. This can be done in one of the following two manners. The first one, which should be very familiar to ActionScript developers is as follows:
player = new Object(); player.name = "John Smith"; player.lives = 5; player.posX = 10; player.posY = -30;
You can also create objects by defining them as a functions as follows:
function player(name, lives, posX, posY) { player.name = name; player.lives = lives; player.posX = posX; player.posY = posY; } var teddyBear = new player("Teddy", 5, 10, 10); console.log(teddyBear.name);
DOM events
Integrating DOM events allows you to use JavaScript to deal with events that occur within HTML documents.
Mouse events
The DOM exposes mouse events for basic user interactions with the mouse pointer. By using the onclick
event parameter within an HTML tag, we can execute JavaScript when a user clicks on a specific element:
<img src="my-image.jpg" id="my-image" onclick="PLACE YOUR JAVASCRIPT HERE">
However, we can also target the element completely from JavaScript and deal with the event handler outside our HTML source code, to keep our project clean and easy to understand:
document.getElementById("my-image").onclick=function() { // Place your JavaScript here... };
Of course, you are not limited to just clicks for mouse events. Events can also be handled for mouse over, mouse out, mouse down, and mouse up. In the examples within this book, we will utilize all of these events as well as methods to extend them even further.