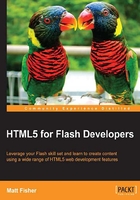
Debugging and output methods
With the popularity of HTML5 and other heavily client-side driven web content, comes the need for a robust developer toolset to allow for easy debugging and testing of web pages. Fortunately, each of the modern browsers have adapted or integrated some very similar setups for doing just this. One of the most important features within this toolset is the JavaScript console. The JavaScript console is to web developers what the Flash Output window is to Flash developers. This is a critically important area to print data from initialized applications or websites and print statements or values specified within the code. In ActionScript, printing data to the output window is accomplished by using the trace()
function. In JavaScript, we utilize the console
object's built-in methods for doing the same. Consider the following example:
function calculateSum(a, b) { sum = a + b; console.log("The sum of " + a + " + " + b + " = " + sum); } calculateSum(2, 3);
Tip
This example can be found within the Console-Example
directory within the Chapter 02_examples
directory.
This code example creates a function in JavaScript to calculate the sum of numbers and calls the method with example parameters to display the output in the browser console. Similar to traces in ActionScript, console integration in JavaScript works behind the scenes, segregated from the actual web page. The primary function of the console is to aid a developer with debugging JavaScript, CSS, or HTML properties during runtime. Not only can the developer console be used for printing data from the application but it can also be used to trigger specific functions in your code without the need for a specific event or interaction to take place.
As important as the console is, the entire user interface and interaction changes depending on what browser is being used to view the document. Therefore, understanding where to find and how to use the console in all popular browsers is an important step in helping you build robust code. Let's quickly run our simple calculate sum example in a couple of the common browsers to see how they handle the output.
Google Chrome
All versions of Google Chrome come packaged with a built-in developer toolset and it can be easily opened by right-clicking on a web page and selecting the Inspect Element option in the dialog box. This will reveal the developer tools window attached to the bottom of the browser window. Selecting the Console tab will display the JavaScript console to view the output from the web page. Opening our JavaScript calculateSum
function example in Chrome with the console open should display something like the following image:

As you can see, the output from the console.log()
call has been displayed along with what file and line number the call was dispatched from. Even from a simple perspective, I am sure you are starting to see how handy this tool could be if you have 100 or even 1000 lines of code in multiple files to deal with. As similar to the output window for traces in ActionScript this tool is, the cherry on top of the pie is its ability to invoke further JavaScript execution directly from the console window. Within the console, we can continue and find the sum of new numbers by calling the calculateSum
function with the necessary values directly from the console.

Some browsers, such as Chrome, even have auto completion feature that expands the text as you type in method or property names, a feature I am sure most Flash developers wished they had within the Flash IDE.
Firebug for Firefox
Since Firefox does not come pre-packed with a robust developer toolset, a common option for web developers is to install the Firebug extension to enable this feature. The extension can easily be added to your Firefox installation in a couple of seconds by visiting http://getfirebug.com. Once installed and activated, right-click anywhere on a page and select Inspect Element with Firebug.

This should all feel pretty familiar to what we did within Chrome. Firebug is a great little project that almost all developers I know utilize. There are a ton of great features in all of these toolsets, many of which we will touch on in this book. Since we have a very simple HTML page open with barely anything in it, now might be a good time to see the UI and output from a more vanilla web page, so feel free to click around and check things out.

Safari
To enable the developer tools in Safari, open the Preferences window and select the Advanced tab. Select the check box at the bottom of the window labeled Show Develop menu in menu bar and then you can close the window.

From this point on, you can right-click, as usual, on any web page and select Inspect Element to display the tools window.

If you were paying attention, you may notice that this console is almost identical to the console within Google Chrome. Of course, it has a command-line integration as we have seen in the other browsers.

Opera
Similar to Google Chrome, the developer tools in Opera can be easily accessed by right-clicking on a web page and selecting Inspect Element. Once the developer tools window opens at the bottom of the browser, select the Console tab to open the developer console. Initially, the console will be blank and clear of any interaction from the web page you are currently viewing.

Rather than having the console always active, Opera has decided to read console commands only when the console is actually open. Therefore, refreshing the page will reveal the console interaction:

Internet Explorer
As of Internet Explorer 9, Microsoft has begun including a developer's toolset directly within the browser. The Developers Tools window can be opened at anytime by pressing F12 while viewing a page. Just like Opera, Internet Explorer requires a page to be refreshed to enable the usage of the console on the active page, as it stays inactive when it is closed.

Of course, just like every other console, we can call our JavaScript methods and variables from the command line.
