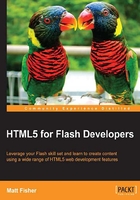
Example JavaScript in action
With all of the JavaScript syntax specifications covered, let's use some of them into a working example and see what happens. Have a look at the following simple HTML document containing JavaScript to sort a randomized array of numbers:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8" /> <title>Insertion Sort - JavaScript Syntax Example</title> <script type="text/javascript"> // Number of elements to sort. elementCount = 10000; // The array which will be sorted. sortlist = new Array(); /** * Called on button click. */ function init() { // Prepare random array for sorting. for(i = 0; i < elementCount; i++) sortlist.push(i); //shuffle(sortlist); sortlist.sort(function() { return 0.5 - Math.random(); }); // Display the random array prior to sorting. console.log(sortlist); // Start a timer. console.time('Iteration Sort Timer'); // Sort the randomized array. insertionSort(sortlist); // Stop the timer. console.log('Sorted ' + elementCount + ' items.'); console.timeEnd('Iteration Sort Timer'); // Display the sorted array. console.log(sortlist); } /** * The popular Insertion Sort algorithm. */ function insertionSort(list) { // It's always smart to only lookup array size once. l = list.length; // Loop over supplied list and sort. for(i = 0; i < l; i++) { save = list[i]; j = i; while(j > 0 && list[j - 1] > save) { list[j] = list[j - 1]; j -= 1; } list[j] = save; } } </script> </head> <body> <p> Click the button below to begin. Be sure to open up your browsers developer console. </p> <button onclick="init()">Start Sorting</button> </body> </html>
This example covers many of the features and syntax specifications of JavaScript that we have just covered. Within our JavaScript block declared in the HTML document head
tag, we have created two functions. The first function is our initiation method to prepare and run the application once it called. The second function contains the popular insertion sort algorithm, which will sort our randomized array of numbers. To enable both functions to use the same variable, we create elementCount
and sortlist
as global variables outside of each function's scope. Within the HTML body
tag is a button
element, which renders a typical form button element on the page and when a user clicks this button, the onclick
handler calls the init
function.
This example isn't flashy by any means but, as I mentioned above, it covers many of the different aspects of the JavaScript syntax specifications.