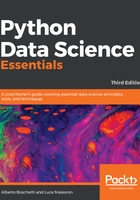
Jupyter magic commands
As a special tool for interactive tasks, Jupyter offers special commands that help to better understand the code that you are currently writing.
For instance, some of the commands are as follows:
- * <object>? and <object>??: This prints a detailed description (with ?? being even more verbose) of <object>
- %<function>: This uses the special <magic function>
Let's demonstrate the usage of these commands with an example. We first start the interactive console with the jupyter command, which is used to run Jupyter from the command line, as shown here:
$> jupyter console
Jupyter Console 4.1.1
In [1]: obj1 = range(10)
Then, in the first line of code, which is marked by Jupyter as [1], we create a list of 10 numbers (from 0 to 9), assigning the output to an object named obj1:
In [2]: obj1?
Type: range
String form: range(0, 10)
Length: 10
Docstring:
range(stop) -> range object
range(start, stop[, step]) -> range object
Return an object that produces a sequence of integers from
start (inclusive)
to stop (exclusive) by step. range(i, j) produces i, i+1, i+2,
..., j-1.
start defaults to 0, and stop is omitted! range(4) produces 0,
1, 2, 3.
These are exactly the valid indices for a list of 4 elements.
When step is given, it specifies the increment (or decrement).
In [3]: %timeit x=100
The slowest run took 184.61 times longer than the fastest.
This could mean that an intermediate result is being cached.
10000000 loops, best of 3: 24.6 ns per loop
In [4]: %quickref
In the next line of code, which is numbered [2], we inspect the obj1 object using the Jupyter command ?. Jupyter introspects the object, prints its details (obj is a range object that can generate the values [1, 2, 3..., 9] and elements), and finally prints some general documentation on the range objects. For complex objects, the usage of ?? instead of ? provides even more verbose output.
In line [3], we use the timeit magic function with a Python assignment (x=100). The timeit function runs this instruction many times and stores the computational time needed to execute it. Finally, it prints the average time that was taken to run the Python function.
We complete the overview with a list of all the possible special Jupyter functions by running the quickref helper function, as shown in line [4].
As you must have noticed, each time we use Jupyter, we have an input cell and, optionally, an output cell if there is something that has to be printed on stdout. Each input is numbered so it can be referenced inside the Jupyter environment itself. For our purposes, we don't need to provide such references in the code of this book. Therefore, we will just report inputs and outputs without their numbers. However, we'll use the generic In: and Out: notations to point out the input and output cells. Just copy the commands after In: to your own Jupyter cell and expect an output that will be reported on the following Out:.
Therefore, the basic notations will be as follows:
- The In: command
- The Out: output (wherever it is present and useful to be reported in this book)
Otherwise, if we expect you to operate directly on the Python console, we will use the following form:
>>> command
Wherever necessary, the command-line input and output will be written as follows:
$> command
Moreover, to run the bash command in the Jupyter console, prefix it with a ! (exclamation mark):
In: !ls
Applications Google Drive Public Desktop
Develop
Pictures env temp
...
In: !pwd
/Users/mycomputer