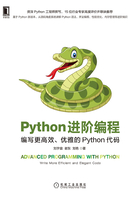
上QQ阅读APP看书,第一时间看更新
1.7 随机数
随机数处理也是实际应用中比较常见的操作,如从一个序列中随机抽取若干元素,或者生成几个随机数,以及生成随机数验证码等。
random模块中有大量的函数用来产生随机数和随机选择元素。如想从一个序列中随机抽取一个元素,可以使用random.choice()函数:
import random values = [1, 2, 3, 4, 5, 6] print(f'random choice from {values} is {random.choice(values)}') print(f'random choice from {values} is {random.choice(values)}') print(f'random choice from {values} is {random.choice(values)}')
执行py文件,输出结果如下:
random choice from [1, 2, 3, 4, 5, 6] is 2 random choice from [1, 2, 3, 4, 5, 6] is 5 random choice from [1, 2, 3, 4, 5, 6] is 3
如果想提取N个不同元素的样本做进一步操作,可使用random.sample()函数:
print(f'random sample 2 from {values} is {random.sample(values, 2)}') print(f'random sample 2 from {values} is {random.sample(values, 2)}') print(f'random sample 3 from {values} is {random.sample(values, 3)}') print(f'random sample 3 from {values} is {random.sample(values, 3)}')
执行py文件,输出结果如下:
random sample 2 from [1, 2, 3, 4, 5, 6] is [5, 6] random sample 2 from [1, 2, 3, 4, 5, 6] is [2, 1] random sample 3 from [1, 2, 3, 4, 5, 6] is [6, 3, 4] random sample 3 from [1, 2, 3, 4, 5, 6] is [3, 4, 6]
如果只想打乱序列中元素的顺序,可以使用random.shuffle()函数:
random.shuffle(values) print(f'random shuffle is:{values}') random.shuffle(values) print(f'random shuffle is:{values}')
执行py文件,输出结果如下:
random shuffle is:[2, 4, 6, 1, 3, 5] random shuffle is:[2, 6, 5, 3, 1, 4]
如果想生成随机整数,可以使用random.randint()函数:
print(f'random.randint(0,10) = {random.randint(0,10)}') print(f'random.randint(0,10) = {random.randint(0,10)}') print(f'random.randint(0,10) = {random.randint(0,10)}')
执行py文件,输出结果如下:
random.randint(0,10) = 9 random.randint(0,10) = 8 random.randint(0,10) = 10
如果想生成0到1范围内均匀分布的浮点数,可以使用random.random()函数:
print(f'random.random() = {random.random()}') print(f'random.random() = {random.random()}') print(f'random.random() = {random.random()}')
执行py文件,输出结果如下:
random.random() = 0.3392503938211737 random.random() = 0.625725029316508 random.random() = 0.3843832669520403
如果要获取N位随机位(二进制)的整数,可以使用random.getrandbits()函数:
print(f'random.getrandbits(200) = {random.getrandbits(200)}')
执行py文件,输出结果如下:
random.getrandbits(200) = 1012257713162841215793585318570079770213053719376074162565721
random模块使用Mersenne Twister算法来生成随机数。这是一个确定性算法,可以通过random.seed()函数修改初始化种子,示例如下:
print(f'random.seed() = {random.seed()}') print(f'random.seed(123) = {random.seed(123)}') print(f"random.seed(b'bytedata') = {random.seed(b'bytedata')}")
执行py文件,输出结果如下:
random.seed() = None random.seed(123) = None random.seed(b'bytedata') = None
除了上述介绍的功能,random模块还包含基于均匀分布、高斯分布和其他分布的随机数生成函数。如random.uniform()函数用于计算均匀分布随机数,random.gauss()函数用于计算正态分布随机数。
random模块中的函数不应该用在和密码学相关的程序中。如果确实需要类似的功能,可以使用ssl模块中相应的函数。如ssl.RAND_bytes()函数可以用来生成一个安全的随机字节序列。