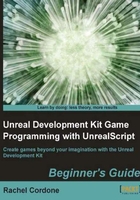
Time for action – Using vectors
Since we already know that an actor's Location is a vector, let's play around with our AwesomeActor's location.
- First we'll declare a vector of our own at the top of our class.
var vector LocationOffset;
- Vectors have their X, Y, and Z values set to 0.0 by default. We'll give ours a new value and add that to our actor's current location in our
PostBeginPlay
function.function PostBeginPlay() { LocationOffset.Z = 64.0; SetLocation(Location + LocationOffset); }
When used as a location, the Z float inside a vector represents the up and down axis. Making the values of Z greater means moving it up, lower or more negative means moving it down. In our example we're going to move the actor up 64 units. We use the function in the second line,
SetLocation
, to tell the game to move ourAwesomeActor
. Since we already know its current location with the Location variable, we just add ourLocationOffset
to move it up 64 units. - There's one thing we need to do before we test this out. When we first created our
AwesomeActor
, we made it invisible in game. Let's change that so we can see what happens. In the default properties of our actor, delete this line:HiddenGame=False
Now our
AwesomeActor
should look like this:class AwesomeActor extends Actor placeable; var vector LocationOffset; function PostBeginPlay() { LocationOffset.Z = 64.0; SetLocation(Location + LocationOffset); } defaultproperties { Begin Object Class=SpriteComponent Name=Sprite Sprite=Texture2D'EditorResources.S_NavP' End Object Components.Add(Sprite) }
- Let's compile and test! In the editor, we can see our
AwesomeActor
still halfway stuck in the floor: - Run the game from the editor, and we can see that our code has worked! The
AwesomeActor
has moved up: - That seems like a bit too much code just to add two vectors together. Luckily, there's a much simpler way to use vectors to do what we want here. Vectors can be created and assigned a value at the same time that we're using it in code, like this:
vect(X value, Y value, Z value)
So if we just wanted to move our actor up 64 units, we could do it all on one line.
- Let's get rid of our
LocationOffset
variable line and change ourPostBeginPlay
to look like this:function PostBeginPlay() { SetLocation(Location + vect(0,0,64)); }
- Compile and test, and we get the same result!
Another useful function we can use with vectors is called VSize. If you remember your old geometry lessons, Pythagoras' Theorem lets us find the hypotenuse of a triangle by using the two sides. We can use a 3D version of the same equation to find the length of our vector.
With that diagram, the length of Vector would be expressed as this:
Length = √(X² + Y² + Z²)
With VSize, all of that is done for us:
Length = VSize(Vector)
So with that in mind, let's find out how far our
AwesomeActor
is from the world origin (0,0,0). - Let's change our
PostBeginPlay
function to this:function PostBeginPlay() { 'log("Distance:" @ VSize(Location)); }
- Compile and test, and the answer shows up in the log!
[0007.88] ScriptLog: Distance: 2085.2571
If we wanted to find out the distance between two actors, we would use their locations like this:
Distance = VSize(A.Location – B.Location);
The order doesn't matter inside the VSize, we could also find the distance like this:
Distance = VSize(B.Location – A.Location);
What just happened?
Vectors are the struct we'll be using the most in UnrealScript, especially since they're used to hold an actor's Location. As we can see from the VSize
and vect
examples, vectors go beyond being just a struct and have their own functions dedicated exclusively to them.
One other variable to discuss also has functions dedicated to it.
Rotators
In the same way vectors are defined as a struct, rotators are as well.
struct immutable Rotator { var() int Pitch, Yaw, Roll; };
Rotators define a rotation in 3D space. To visualize it it helps to think of an airplane flying in the sky.

Pitch would be the airplane tilting forwards and backwards to climb or descend. Roll would be the plane tilting sideways, and Yaw would be the plane rotating horizontally, like a spinning frisbee.
In addition to a Location vector, every actor in the game has a rotator variable called Rotation that tells the game what direction it's facing. Rotating a wall in the editor changes that static mesh's rotation value. The player's view rotation is held in a rotator. They're not used as often as vectors, but they're obviously still important. Let's take a look at them.