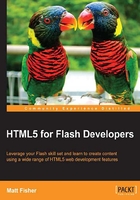
Audio and video playback control
As we saw in the last chapter, integrating audio and video assets with basic controls into and HTML5 document is extremely easy. But if you intend to use your multimedia in other forms than just a straightforward video playback element, you will need to understand the properties available for custom playback code integration.
Preloading
By default, when an audio or video element is displayed in a HTML5 document, the source asset declared within it will be preloaded to allow for instantaneous playback when the user initiates the player. Assets will be preloaded only as far as the browsers deems necessary to enable fluid uninterrupted playback. To override this setting, we can use the preload
parameter within the audio element to declare what we would like to be preloaded when a user views our page.
Setting the preload parameter to auto
will preload the entire audio upon page load and could be a useful addition to any audio you are almost certain a user will watch at some point after the page loads. With the preload
parameter set, our audio element would look something like the following:
<audio controls preload="all"> <source src="my-audio .mp3" type="audio/mpeg"> <source src="my-audio.ogg" type="audio/ogg"> Your browser does not support the audio element. </audio>
Aside from preloading everything, we can also preload absolutely nothing by setting preload="none"
rather than auto
. Removing preloading from audio will allow users to surf your pages without the need for unnecessary audio downloads but will result in longer loading times for audio to player once the user initiates the audio playback. Finally we can also just load audio metadata when preloading by setting preload="metatdata"
. This will allow the audio element to view what data it is about to load, which can be very useful when dynamically adding audio into an audio element and requiring the need to verify if it is fit for playback before attempting to do so.
Autoplay
As described in Chapter 2, Preparing for the Battle, with the autoplay
setting appended to a video element, the video will begin to play the moment it is able to do so without the need to stop the video for further buffering. Unlike many of the other element parameters in HTML, the autoplay
parameter does not require a value. So just appending autoplay
to the element will be enough to do the job. It is worth keeping in mind that the autoplay
setting will be ignored when loaded on almost all mobile browsers. Mobile browsers tend to ignore this setting in an attempt to conserve bandwidth on a wireless connection.
Looping
With the loop setting appended to the audio element, the video will restart every time is finishes. Like the autoplay
parameter, the loop
parameter does not require a value. If you only wanted a video to loop a specific number of times, you can either watch how many times its loops with the loop
parameter set and then remove it when necessary, or control the entire playback from JavaScript to control the loop count without the loop parameter in the video element.
Sound effects
Playing a sound effect at a specific moment can be accomplished in a number of ways with the use of the HTML5 audio element and JavaScript. In its simplest form, playing sound effects can be implemented as done the following code example:
<body> <audio src="audio/ping.mp3" preload="auto" id="audio-ping"> </audio> <script> window.addEventListener("load", init, false); function init() { window.addEventListener( 'mousedown', onMouseDown, false ); } function onMouseDown(e) { document.getElementById('audio-ping').play(); } </script> </body>
When the audio element is created inside the HTML document body, we set the preload="auto"
which will make sure the audio is preloaded completely as soon as possible. We do this so there is no latency when the sound is needed during the effect event. The audio element is also given an ID to enable referencing in JavaScript a couple line down. With the window load event listener, we wait for page load then apply an event listener to any mousedown
event anywhere in the browser window. When this fires, we select our audio element by ID and call the built in play()
method resulting in the audio playback on every click of the browser window.
Media playback manipulation
Aside from the play(
) method in the preceding example, JavaScript has the accessibility to control much more of the audio and video element directly. Audio volume can be set as a value between 0
and 1
as shown in the following example:
document.getElementById('audio-ping').volume = 0.5; // Set the volume to 50%
We can also gather all the stats on the element by utilizing the following exposed objects within it:
var media = document.getElementById('audio-ping'); media.seekable.start(); // Start time (seconds) media.seekable.end(); // End time (seconds) media.currentTime = 20; // Seeks playback to 20 seconds // Total amount of seconds the playback has displayed media.played.end();