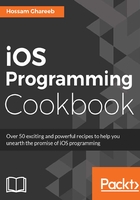
上QQ阅读APP看书,第一时间看更新
How to do it...
Now, let's imagine that we are working on a game, and you have different types of monsters, and based on the type of monster, you will define power or the difficulty of the game. In that case, you have to use enum to create a monster type with different cases, as follows:
- Type the following code to create enum with name Monster:
enum Monster{ case Lion case Tiger case Bear case Crocs } enum Monster2{ case Lion, Tiger, Bear, Crocs }
- Use the '.' operator to create enums variables from the previously created enum:
var monster1 = Monster.Lion let monster2 = Monster.Tiger monster1 = .Bear
- Use the switch statement to check the value of enum to perform a specific action:
func monsterPowerFromType(monster:Monster) ->Int { var power = 0 switch monster1 { case .Lion: power = 100 case .Tiger: power = 80 case .Bear: power = 90 case .Crocs: power = 70 } return power } let power = monsterPowerFromType(monster1) // 90 func canMonsterSwim(monster:Monster) ->Bool{ switch monster { case .Crocs: return true default: return false } } let canSwim = canMonsterSwim(monster1) // false