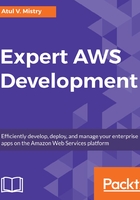
上QQ阅读APP看书,第一时间看更新
Configuring an SDK as a Maven dependency
Please perform the following steps to configure AWS SDK as Maven dependency.
- To add AWS SDK for Java in your project, you need to add the dependency to the pom.xml file. From SDK version 1.9.*, you can import single or individual components. If you want to add the entire SDK as a dependency, add the following code in the <dependency> tag in the pom.xml file:
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-java-sdk</artifactId>
<version>1.11.106</version>
</dependency>
- If you are using SDK Version 1.9.* or above, you can import many individual components, such as EC2, S3, CodeCommit, or CodeDeploy:
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-java-sdk-s3</artifactId>
</dependency>
- After setting up the pom.xml file, you can build your project with the mvn package command. It will generate a Java Archive (JAR) file in the target directory after successful execution:

- Now you need to add the following code in the pom.xml file to connect with the AWS SDK. You have to mention your main Java class under the Configuration | mainClass tag. We will create the S3MavenExample.java file in the next step:
<build>
<resources>
<resource>
<directory>${env.HOME}/.aws/</directory>
</resource>
</resources>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>exec-maven-plugin</artifactId>
<version>1.2.1</version>
<executions>
<execution>
<goals>
<goal>java</goal>
</goals>
</execution>
</executions>
<configuration>
<mainClass>com.packt.example.S3MavenExample</mainClass>
</configuration>
</plugin>
</plugins>
</build>
- Let's create the S3MavenExample.java file in the com/packt/example package. We are going to create an S3 bucket as per the specific region with a random number generator, prefix it with s3-maven-bucket-, and then delete the bucket:
import java.util.UUID;
import com.amazonaws.regions.Region;
import com.amazonaws.regions.Regions;
import com.amazonaws.services.s3.AmazonS3;
import com.amazonaws.services.s3.AmazonS3Client;
- You have imported UUID to generate the pseudo random number. You are importing Region and Regions to create the bucket in a specific region, and AmazonS3 and AmazonS3Client to access the AWS S3 services:
AmazonS3 s3 = new AmazonS3Client();
Region s3Region = Region.getRegion(Regions.AP_SOUTHEAST_1);
s3.setRegion(s3Region);
- Here you are creating an S3 client and specifying the specific region where it will create the bucket:
If you do not specify any region, it will create it at US East (N. Virginia). This means the default region is US East (N. Virginia).
String bucketName = "s3-maven-bucket-" + UUID.randomUUID();
- Here you are creating the s3-maven-bucket- prefix with some random UUID:
s3.createBucket(bucketName);
- To create the bucket, you can use createBucket() method. You have to pass the bucket name as a parameter in this method:
s3.deleteBucket(bucketName);
- To delete the bucket, you can use the deleteBucket() method. You have to pass the bucket name as a parameter in this method. After creating the Java file, execute the following command:
mvn clean compile exec:java
It will create and delete the bucket as per the specified regions:

If you have completed this step and you can see the creation and deletion of the bucket, it means you have successfully completed AWS SDK for Java using Maven in your project.