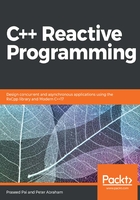
Using Lambdas
Now, let's see the usefulness of Lambda expressions for multithreading. In the following code, we are going to create five threads and put those into a vector container. Each thread will be using a Lambda function as the initialization function. The threads initialized in the following code are capturing the loop index by value:
int main() { std::vector<std::thread> threads; for (int i = 0; i < 5; ++i) { threads.push_back(std::thread( [i]() { std::cout << "Thread #" << i << std::endl; })); } std::cout << "nMain function"; std::for_each(threads.begin(), threads.end(), [](std::thread &t) { t.join(); }); }
The vector container threads store five threads that have been created inside the loop. They are joined at the end of the main() function once the execution is over. The output for the preceding code may look as follows:
Thread # Thread # Thread # Thread # Thread #
Main function
0
4
1
3
2
The output of the program could be different for each run. This program is a good example to showcase the non-determinism associated with concurrent programming. In the following section, we will discuss the move properties of a std::thread object.