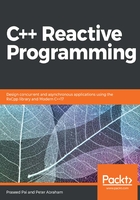
Thread launch
In the previous example, we saw that the initialization function is passed as an argument to the std::thread constructor, and the thread gets launched. This function runs on its own thread. The thread launch happens during the thread object's construction, but the initialization functions can have other alternatives as well. A function object is another possible argument in a thread class. The C++ standard library ensures that the std::thread works with any callable type.
The modern C++ standard supports threads to be initialized through:
- Function pointers (as in the previous section)
- An object that implements the call operator
- Lambdas
Any callable entity is a candidate for initializing a thread. This enables the std::thread to accept a class object with an overloaded function call operator:
class parallel_job { public: void operator() () {
some_implementation(); } }; parallel_job job; std::thread t(job);
Here, the newly created thread copies the object into its storage, hence the copy behavior must be ensured. Here, we can also use std::move to avoid problems related to copying:
std::thread t(std::move(job));
If you pass temporary (an rvalue) instead of a function object, the syntax is as follows:
std::thread t(parallel_job());
This code can be interpreted by the compiler as a declaration of a function that accepts a function pointer and returns a std::thread object. However, we can avoid this by using the new uniform initialization syntax, as follows:
std::thread t{ parallel_job() };
An extra set of parenthesis, as given in the following code snippet, can also avoid the interpretation of std::thread object declaration into a function declaration:
std::thread t((parallel_job()));
Another interesting way to launch a thread is by giving the C++ Lambdas as an argument into a std::thread constructor. Lambdas can capture local variables and thus avoid unnecessary usage of any arguments. Lambdas are very useful when it comes to writing anonymous functions, but that doesn't mean that they should be used everywhere.
The Lambda function can be used along with a thread declaration as follows:
std::thread t([]{ some_implementation(); });