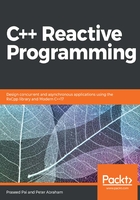
Lambda functions
One of the major additions to the C++ language is Lambda functions and Lambda expressions. They are anonymous functions that the programmer can define at the call site to perform some logic. This simplifies the logic and code readability also increases in a remarkable manner.
Rather than defining what a Lambda function is, let us write a piece of code that helps us count the number of positive numbers in a vector<int>. In this case, we need to filter out the negative values and count the rest. We will use an STL count_if to write the code:
//LambdaFirst.cpp
#include <iostream>
#include <iterator>
#include <vector>
#include <algorithm>
using namespace std;
int main() {
auto num_vect =
vector<int>{ 10, 23, -33, 15, -7, 60, 80};
//---- Define a Lambda Function to Filter out negatives
auto filter = [](int const value) {return value > 0; };
auto cnt= count_if(
begin(num_vect), end(num_vect),filter);
cout << cnt << endl;
}
In the preceding code snippet, the variable filter is assigned an anonymous function and we are using the filter in the count_if STL function. Now, let us write a simple Lambda function that we will specify at the function call site. We will be using STL accumulate to aggregate the values inside a vector:
//-------------- LambdaSecond.cpp
#include <iostream>
#include <iterator>
#include <vector>
#include <algorithm>
#include <numeric>
using namespace std;
int main() {
auto num_vect =
vector<int>{ 10, 23, -33, 15, -7, 60, 80};
//-- Define a BinaryOperation Lambda at the call site
auto accum = std::accumulate(
std::begin(num_vect), std::end(num_vect), 0,
[](auto const s, auto const n) {return s + n;});
cout << accum << endl;
}