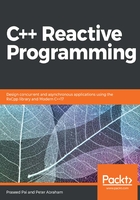
Rvalue references
If you have been programming in C++ for a long time, you might be familiar with the fact that C++ references help you to alias a variable and you can do assignment to the references to reflect the mutation in the variable aliased. The kinds of reference supported by C++ were called lvalue references (as they were references to variables that can come in the left side of the assignment). The following code snippets show the use of lvalue references:
//---- Lvalue.cpp
#include <iostream>
using namespace std;
int main() {
int i=0;
cout << i << endl; //prints 0
int& ri = i;
ri = 20;
cout << i << endl; // prints 20
}
int& is an instance of lvalue references. In Modern C++, there is the notion of rvalue references. rvalue is defined as anything that is not an lvalue, the kind of stuff that can appear on the right side of the assignment. In classic C++, there was no notion of an rvalue references. Modern C++ introduced it:
///---- Rvaluref.cpp
#include <iostream>using namespace std;
int main() {
int&& j = 42;int x = 3,y=5; int&& z = x + y; cout << z << endl;
z = 10; cout << z << endl;j=20;cout << j << endl;
}
Rvalue references are indicted by two &&. The following program will clearly demonstrate the use of rvalue references while invoking functions:
//------- RvaluerefCall.cpp
#include <iostream>
using namespace std;
void TestFunction( int & a ) {cout << a << endl;}
void TestFunction( int && a ){
cout << "rvalue references" << endl;
cout << a << endl;
}
int main() {
int&& j = 42;
int x = 3,y=5;
int&& z = x + y;
TestFunction(x + y ); // Should call rvalue reference function
TestFunction(j); // Calls Lvalue Refreence function
}
The real power of rvalue references is visible in the case of memory management. The C++ programming language has got the notion of Copy constructor and Assignment operators. They mostly copy the source object contents. With the help of rvalue references, one can avoid expensive copying by swapping pointers, as rvalue references are temporaries or intermediate expressions. The following section demonstrates this.