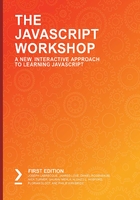
Making XHR Requests
A fundamental functionality of modern web pages and applications is requesting additional resources or data from remote servers. Every browser provides interfaces to execute these so-called XHR requests. Those interfaces can be used from JavaScript. As we can see in the following code examples, jQuery, compared to vanilla.js, lets us write clean and self-explanatory code:
// Vanilla.js
const request = new XMLHttpRequest();
request.open("POST", "/example/api", true);
request.onreadystatechange = function() {
if (request.readyState != 4 || request.status != 200) return;
console.log("Successful XHR!");
};
request.send("example=payload");
In comparison to the preceding snippet, the code for making calls to a server is much clear and readable in jQuery. It is more readable in the sense that it is very clear and understandable regarding what exactly the function needs as parameters and what it is going to do. Let's have a look at a POST Ajax request to the /example/api URL with a specified payload data and also a function that gets triggered when the request is successful:
// jQuery
$.ajax({
type: "POST",
url: "/example/api",
data: "example=payload",
success: function() {
console.log("Successful XHR!");
}
});
Note
jQuery assigns itself to the $ variable. Hence, in the code examples,$.functionName could be replaced with jquery.functionName.
We could go on and show you more use cases where jQuery gets us faster to our goal than raw JS would. Instead, in the following exercises, we will use the library and gain some first-hand experience with it. Specifically, we will be writing code to handle a button-click event, once with jQuery and once with plain JavaScript.
Note
The dev tools in all modern major browsers have adapted to $, but only as a wrapper for document.querySelector.
Exercise 4.02: Handling Click Events with jQuery
In this exercise, we will identify how jQuery can help us react to events that get propagated when the target (in our case, a button) gets clicked. Let's get started:
- Create a new HTML file including a button tag with the ID exampleButton. This button will be our target:
<html>
<body>
<button id="exampleButton">Click me.</button>
</body>
</html>
- Find the latest jQuery CDN URL on cdnjs.com.
- Read the jQuery documentation for .on()(https://api.jquery.com/on/) erytion:
Figure 4.7: jquery.com Documentation for .on()
- Load in the CDN URL:
<html>
<head>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.
js"></script></head>
<body>
<button id="exampleButton">Click me.</button>
</body>
</html>
- Create a script tag containing code that logs a Hello World message to the console when you click the button:
<html>
<head>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.
js"></script></head>
<body>
<button id="exampleButton">Click me.</button>
<script type="text/javascript">
$('#exampleButton').on('click', function() {
console.log('Hello World')
})
</script>
</body>
</html>
- Make sure you place the script tag after the button tag.
- Open the HTML in your browser and open the dev tool console.
- Press the Click me. button and verify that it prints Hello World to the console:
Figure 4.8: Hello world output using jQuery click events
In this exercise, you handled an event that got fired by the browser on a button click using jQuery. The handler you implemented prints Hello World to the browser's console as soon as the click me button is pressed.
You also saw how easy it is to use a library such as jQuery to do work that, otherwise, you would need to do manually.
Handling a click event, however, is not particularly hard to do in vanilla.js either, as you'll see in the next exercise.
Exercise 4.03: Handling the Same Event with Vanilla.js
In contrast to the previous exercise, this one demonstrates how to create a handler that gets triggered on a click event using plain JavaScript. Let's get started:
- Create a new HTML file that includes a button tag with the ID exampleButton:
<html>
<body>
<button id="exampleButton">Click me.</button>
</body>
</html>
- Create a script tag containing vanilla.js code that logs a Hello World message to the dev tools console when you click the button. addEventListener is a vanilla API provided to us by the browser. It takes eventType and handlerFunction as parameters:
<html>
<body>
<button id="exampleButton">Click me.</button>
<script type="text/javascript">
const button = document.querySelector('#exampleButton')
button.addEventListener('click', function() {
console.log('Hello World')
})
</script>
</body>
</html>
Again, make sure you place the script tag after the button tag.
- Open the HTML in your browser and open the dev tools console. Press the "Click me." button and verify that it prints "Hello World" to the console:
Figure 4.9: Hello World output using Vanilla.js
In the previous two exercises, we added an event listener to a button. We did so once with the help of jQuery and the other time with no external code and instead used the native APIs that the browser provided us with.
UI Animation Using jQuery
In addition to the use cases for jQuery that we have seen in the code examples in the Manipulating the DOM and Making XHR Requests sections and in Exercise 4.02: Handling Click Events with jQuery, there is another important functionality that jQuery provides us with: animating the user interface (UI).
Animations contribute to a more engaging website and can mean that your users enjoy the experience of using your application more. Often reactions to user input are animated to highlight the fact that the interaction has been acknowledged or that something has changed. For example, appearing elements could be animated or placeholders inside of input fields. Proceed with the following exercise to implement the former UI animation example yourself.
Exercise 4.04: Animating a "Peek-a-boo" on Button Click
In this exercise, you will build on the knowledge you have gained regarding how to handle events using jQuery. The relevant part, however, will be animating an element on the page.
Whenever the "Peek…" button is clicked, the …a-boo headline will show up. Let's get started:
- Create a new HTML file that includes a button tag with the ID Peek..., a headline with the ID …a-boo, and a display: none style attribute:
<html>
<head></head>
<body>
<button id="peek">Peek...</button>
<h1 id="aboo" style="display: none;">...a-boo</h1>
</body>
</html>
- Load the latest jQuery CDN URL, from cdnjs.com (see Exercise 2, Handling Click Events with jQuery, step 2), inside a script tag:
<html>
<html>
<head>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquer
y.min.js"></script>
</head>
<body>
<button id="peek">Peek...</button>
<h1 id="aboo" style="display: none;">...a-boo</h1>
</body>
</html><head>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquer
y.min.js"></script>
</head>
<body>
<button id="peek">Peek...</button>
<h1 id="aboo" style="display: none;">...a-boo</h1>
</body>
</html>
- Create a script tag containing the code to select the peek button and add an onClick event listener:
<html>
<head>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.
js"></script></head>
<body>
<button id="peek">Peek...</button>
<h1 id="aboo" style="display: none;">...a-boo</h1>
<script type="text/javascript">
const peekButton = $(‚#peek');
peekButton.on('click', function() {});
</script>
</body>
</html>
- Inside the new script tag, write additional code to select the aboo headline and use the jQuery.fadeToggle function to animate the headline so that it's fading in and fading out:
<html>
<head>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.
js"></script></head>
<body>
<button id="peek">Peek...</button>
<h1 id="aboo" style="display: none;">...a-boo</h1>
<script type="text/javascript">
const peekButton = $('#peek');
const abooHeadline = $('#aboo');
peekButton.on('click', function() {
abooHeadline.fadeToggle();
});
</script>
</body>
</html>
- Open the HTML page in your browser and click the peek button.
- You should see the aboo headline fading in and fading out whenever you click the peek button:
Figure 4.10: Animated output using the Click button
In this exercise, you used jQuery to execute yet another type of task in the browser. Animations in UIs can be as simple as our fading example, but they can also be very complex when building games or creating 3D animations.
By now, you have an idea of what jQuery, and also other libraries or frameworks, can help you do. In the next section, we will explore why and when it may be wiser to renounce external source code.