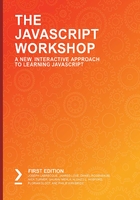
HTML Element Manipulation with JavaScript
We have alluded to the ability of JavaScript to directly manipulate elements within an HTML document a number of times. We'll now go ahead and see firsthand exactly how the language can be leveraged to perform this sort of manipulation.
Before moving on to the activity, there are a couple of concepts to understand so that you know how HTML elements function. As you've seen through example, elements in HTML generally consist of an opening and closing tag, within which there is often text data. If you think of a <p> tag or paragraph element in HTML, the text within that element, between the opening and closing tags, is the text displayed to the user.
If we need to address a specific HTML element to manipulate, the best way to do so is with the getElementById() JavaScript method. Of course, the element in question must contain an ID attribute for this to function as expected.
For example, maybe we have a simple HTML unordered list that contains a set of three list items:
<ul id="frameworks">
<li>Angular</li>
<li>Vue</li>
<li>React</li>
</ul>
What if we wanted to manipulate this list through code? We can do so with some simple JavaScript. First, we have to store a reference to our list itself. Since the list element has an id attribute with a value of frameworks, we can store a reference to this element using the following code:
var frameworksList = document.getElementById('frameworks');
With a reference created, we can now easily create and add a new child element:
var newFramework = document.createElement('li');
newFramework.innerText = "Apache Royale";
frameworksList.appendChild(newFramework);
We can even adjust the style properties of our newly added element:
frameworksList.lastChild.style.color = "crimson";
getElementById() is a JavaScript function that returns an element that has been granted a certain ID attribute and is an exact match for the specific string. An HTML element ID attribute must be unique.
Once we have a reference to any element in JavaScript through the use of getElementById(), we can then get a reference to its child elements through the children.length child attribute and finally get a count of how many child elements exist by invoking the length property.
Additionally, any attributes that are defined as part of these elements can also be manipulated with JavaScript code. In this activity, we'll make adjustments to the style attribute—effectively modifying the visual appearance of the specific element content.
In this section, we've seen how to directly manipulate HTML elements and associated attributes using pure JavaScript code. You should now have a good idea of what this language is capable of.
Activity 2.01: Adding and Modifying an Item to/in the To-Do List
In this activity, we will be examining the beginnings of a small web-based view that is meant to list a set of to-do items. You need to create some boilerplate for the following code, which is currently static HTML and CSS with a placeholder <script> tag. You then need to add an item, Research Wines, to the to-do list and change the font color of the newly added item to crimson. Lastly, you need to verify the order that this code will be executed in.
This activity will help cement the relationship that exists between HTML, CSS, and JavaScript. The following code shows how JavaScript can impact the visual display styles and even the elemental content of HTML nodes:
activity.html
1 <!doctype html>
2 <html lang="en">
3 <head>
4 <meta charset="utf-8">
5 <title>To-Do List</title>
6 <link href=https://fonts.googleapis.com/css?family=Architects+Daughter|Bowlby+One+SC
rel="stylesheet">
7 <style>
8 body {
9 background-color:rgb(37, 37, 37);
10 color:aliceblue;
11 padding:2rem;
12 margin:0;
13 font-family: 'Architects Daughter', cursive;
14 font-size: 12px;
15 }
The full code is available at: https://packt.live/2Xc9Y4o
The steps for this activity are as follows:
- You will create the HTML file yourself and paste in the preceding boilerplate HTML code to get started. This file conforms to a standard HTML document targeting the HTML5 specification. It is structured with a <head> tag and a <body> tag, and nested within the <body> tag exists the visual elements and the data a user would be able to see within their web browser. Additionally, within this file, you will see a set of CSS style rules within the <style> tags, which provide visual HTML elements with some additional specific colors, fonts, and sizes. The CSS refers to additional stylistic font information being loaded from remote Google Fonts (https://fonts.google.com/) services.
- Assign an ID to our list in order to identify it via code.
- Create a new variable and use the ID to address this element directly by the use of the getElementById() method.
- Create a new HTML list item element.
- Populate the list item with a data value.
- Add it to the visual document by appending it to a chosen parent container that already exists.
- Change the color of the existing element.
- Verify the execution order of our commands by counting the initial number of list items.
- Then, count the final number of list items after code execution.
- Refresh the browser view and watch the console.
Note
The solution to this activity can be found on page 712.